namespace
#include <include/spice/noise.hpp>
noise Contents
- Reference
This namespace exports various noise generation algorithms.
Functions
-
template<typename T>void salt_and_pepper(image<T>& source, float density)
-
template<typename T, typename Op = std::plus<T>>void uniform(image<T>& source, T const& min, T const& max, Op operation = Op())
-
template<typename T, typename Op = std::plus<T>>void gaussian(image<T>& source, T const& mean, T const& sigma, Op operation = Op())
Function documentation
template<typename T>
void spice:: noise:: salt_and_pepper(image<T>& source,
float density)
Parameters | |
---|---|
source | The image to modify |
density | The probability of a pixel being either white or black |
Adds salt and pepper noise to the input image.
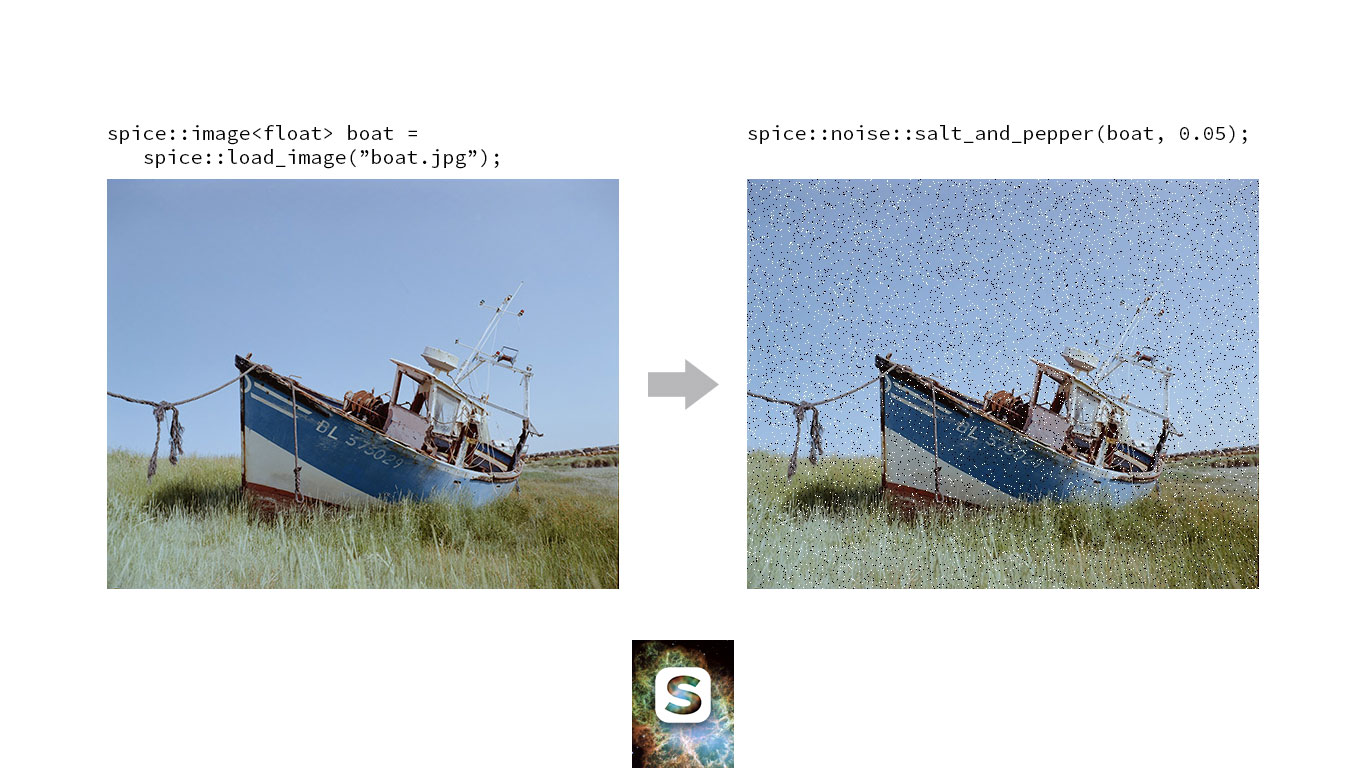
template<typename T, typename Op = std::plus<T>>
void spice:: noise:: uniform(image<T>& source,
T const& min,
T const& max,
Op operation = Op())
Parameters | |
---|---|
source | The image to modify |
min | The minimum noise value |
max | The maximum noise value |
operation | The with which to combine the noise with the image. Please note that the image element will be the first operand and the noise sample the second (i.e. element = operation(element, noise ). |
Adds uniform noise to the input image.
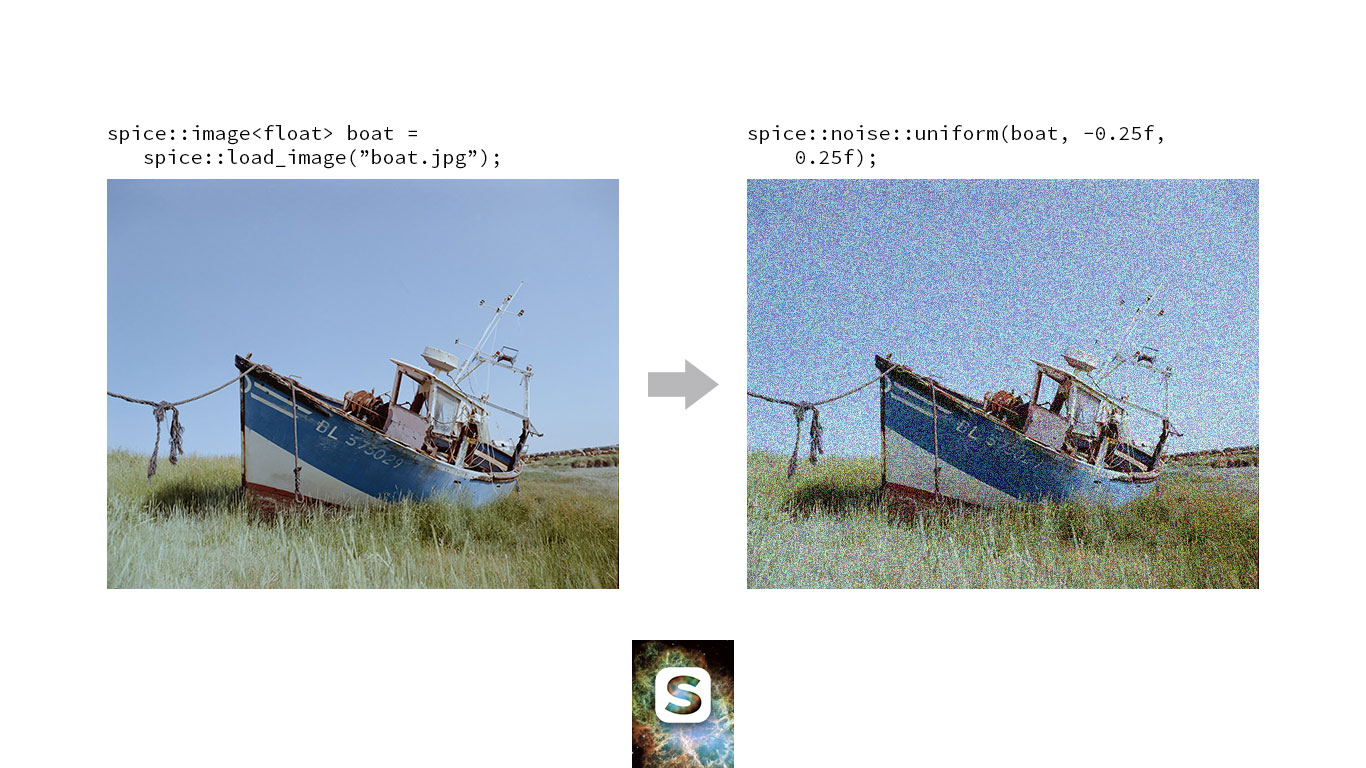
template<typename T, typename Op = std::plus<T>>
void spice:: noise:: gaussian(image<T>& source,
T const& mean,
T const& sigma,
Op operation = Op())
Parameters | |
---|---|
source | The image to modify |
mean | The mean of the noise distribution |
sigma | The standard deviation |
operation | The operation with which to combine the noise with the image. Please note that the image element will be the first operand and the noise sample the second (i.e. element = operation(element, noise ). |
Adds gaussian noise to the input image.
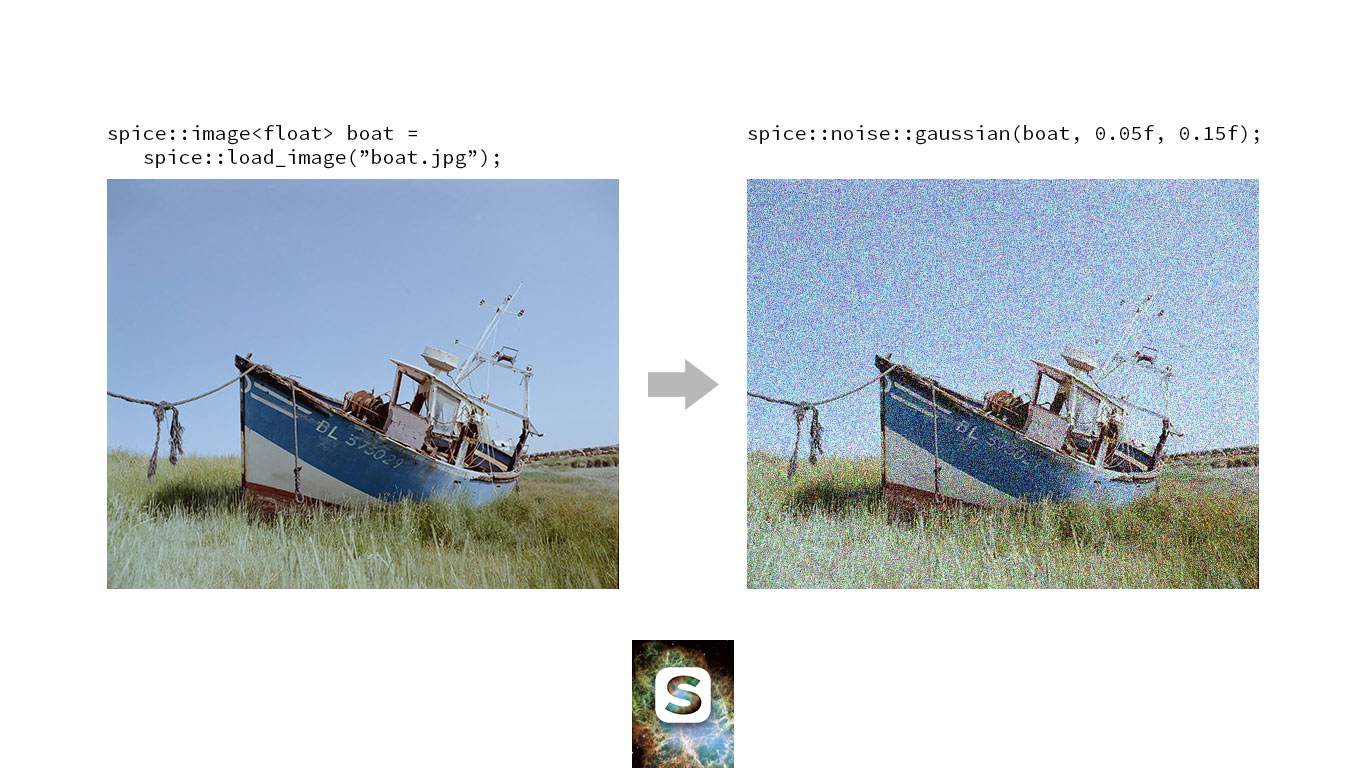